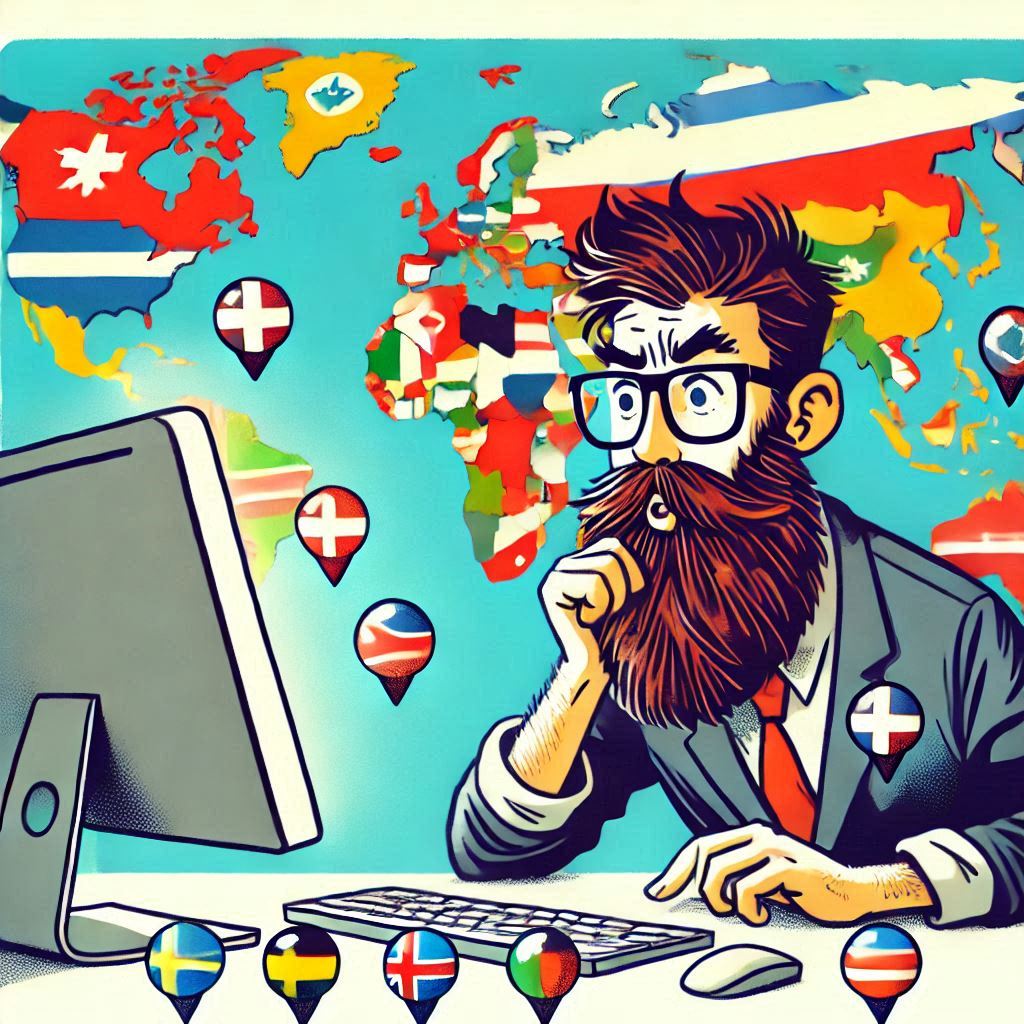
This article will show you how to export named locations in Entra ID using PowerShell. A named location in Conditional Access allows you to label specific IP address ranges or geographic locations that you want to include or exclude in your access policies. This feature lets you control access to your cloud apps based on a user’s network location.
In Entra ID, there isn’t an option to directly export these locations, and copying and pasting from the list can be cumbersome. Therefore, I’ve created a quick script to export the list into a .CSV file which will also include the full country name.
Overview
This script will connect to Microsoft Graph PowerShell, it will require Policy.Read.All. You will need to request consent if you do not have full access. You will also need to get your Entra ID Tenant ID.
PowerShell Script
The PowerShell script is available for download on GitHub or you can copy the script below.
# PowerShell Script to Export Named Location Countries to CSV
# This script will output the 2 letter country code as the full country name
# Created by Wilcox Yuen - Sandbox IT Solutions https://www.sandboxitsolutions.com
# Install the Microsoft Graph module if not already installed
# Install-Module -Name Microsoft.Graph -Force
# Connect to Microsoft Graph
Connect-MgGraph -Scopes "Policy.Read.All" -NoWelcome -TenantId "<your-tenant-id>"
# Get all cultures to map country codes to full names
$cultures = [System.Globalization.CultureInfo]::GetCultures([System.Globalization.CultureTypes]::SpecificCultures)
# Create a hashtable to store country codes and full names
$countryMapping = @{}
# Populate the hashtable with country codes and full names
$cultures | ForEach-Object {
$regionInfo = New-Object System.Globalization.RegionInfo $_.Name
if (-not $countryMapping.ContainsKey($regionInfo.TwoLetterISORegionName)) {
$countryMapping[$regionInfo.TwoLetterISORegionName] = $regionInfo.EnglishName
}
}
# Get the named location
$GetLocation = Get-MgIdentityConditionalAccessNamedLocation -Filter "DisplayName eq 'Named Location goes here'"
# Get the list of country codes from the named location and sort them
$countries = $GetLocation.AdditionalProperties.countriesAndRegions | Sort-Object
# Map the country codes to full names and output a custom object
$fullCountryNames = $countries | ForEach-Object {
[PSCustomObject]@{
CountryCode = $_
CountryName = $countryMapping[$_]
}
}
# Export the full country names to a CSV file
$fullCountryNames | Export-Csv -Path "c:\temp\Sandbox-CountriesListWithFullNames.csv" -NoTypeInformation
How Do I Get my Entra ID Tenant ID?
- Go to https://portal.azure.com.
- Go to Entra ID > Overview and the Tenant ID will be displayed. You can click the copy button to copy.
Before Running the PowerShell Script
There will be a couple of items to change in the PowerShell script before you run it.
- Replace the tenant ID on line 10 with your Tenant ID.

- Edit line 27 and change the named location. An example of my named location is “Blacklisted Countries”.

- Edit line 42 and change the path and filename to your liking.

Results
When you connect for the first time, it will prompt for consent. If you have the correct permissions, you can accept the consent on behalf of your organization.
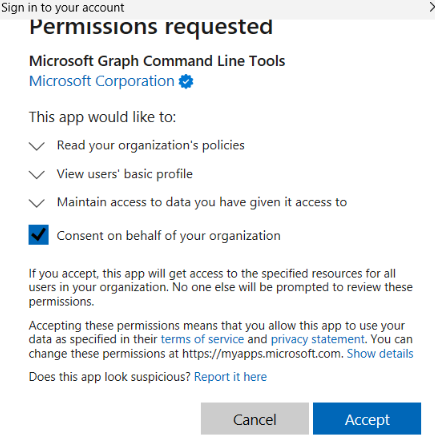
The CSV file will look like this:
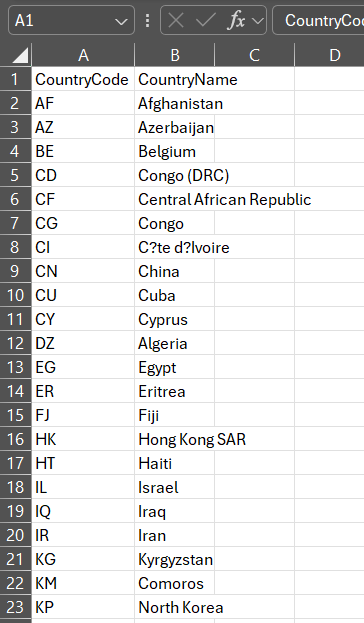